This guide will show you how to check the current location of a local player's ZEPETO character within ZEPETO World.
The location information is stored in the transform object's position, so it is important to access the ZEPETO player's transform to find their location.
STEP 1 : Writing the Script
- Select the [+] menu in the top left corner of the [Project] panel or choose Assets > Create > ZEPETO > TypeScript.
- Rename the added script to
GetPosition
and write a sample script as follows.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
import { ZepetoPlayers, ZepetoCharacter } from 'ZEPETO.Character.Controller';
import { Text } from 'UnityEngine.UI';
import { WorldService } from 'ZEPETO.World';
export default class GetPosition extends ZepetoScriptBehaviour {
public debugText: Text;
private _zepetoCharacter: ZepetoCharacter;
Start() {
ZepetoPlayers.instance.OnAddedLocalPlayer.AddListener(() => {
// Get the ZepetoCharacter of the local player using their userId.
this._zepetoCharacter = ZepetoPlayers.instance.GetPlayer(WorldService.userId).character;
})
}
Update() {
// Update the debug text with the current player's position.
this.debugText.text = "Current Player Position : " + this._zepetoCharacter.transform.position.ToString();
}
}
Caution
To use the
GetPlayer()
function in a multiplayer environment, you must use sessionId as a parameter.
Please refer to the following guide. [Zepeto Players]
- Add a GameObject to the Scene and rename it to
CharacterPosition
.- Add the written
GetPosition
ZEPETOScript, and connect a Button UI to the Property in the ZEPETO Script Inspector window by simply dragging and dropping it from the Hierarchy window.
- Add the written
STEP 2 : Run
- When you press the Play button, you can check the character's real-time location through the Text UI.
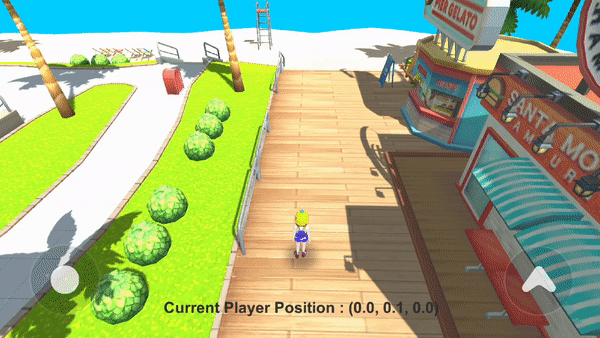