You can send Direct Messages to other users within ZEPETO World using the Send Direct Message(DM) API.
When using the Direct Message (DM) sending API in a launched world, a link to enter the world is automatically provided at the bottom of the message, making it good for promoting the world.
Please be sure to follow the precautions when using.
Install
Window → Package Manager → ZEPETO.World Package Please install version 1.21.14 or higher first.
Afterward, please install the ‘ZEPETO.Module’ package with a version of 1.0.7 or higher.
Ensure that the ZEPETO app is running the version 3.46.000 or higher.
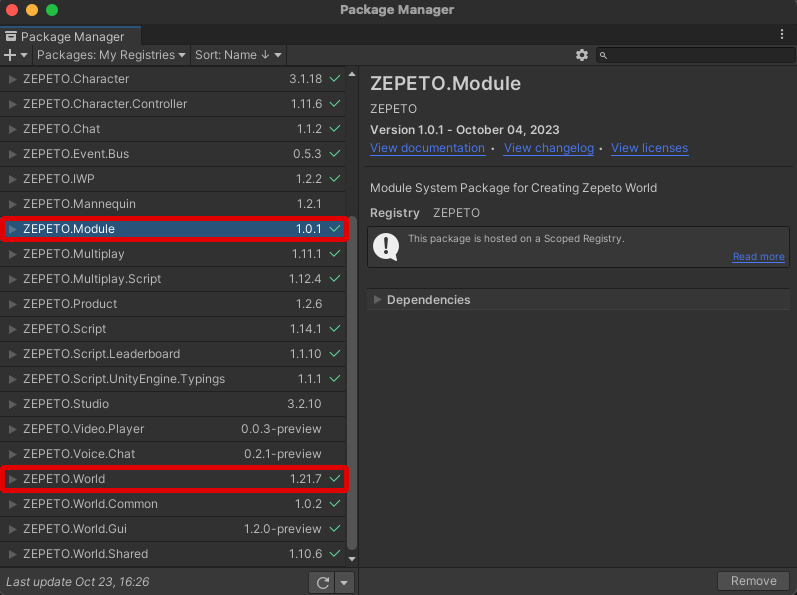
Send Direct Message API
- To use the Send DM API, add the import statement below.
import { SocialService } from 'ZEPETO.Module.Social';
Send DM core function
- Declaration
- public static OpenPopupDirectMessage($sendMessage: string, $userIds?: string[], $onComplete?: System.Action$1):void;
- Parameters
parameter | Description |
---|---|
sendMessage | This is a required parameter. This is the body of the message you want to send. If it is blank or empty, the transmission will be treated as a failure. |
userIds | This is an optional parameter. If you pass userIds - The selection UI is configured with the corresponding user ID list. When userIds are not passed or an empty list is passed - The selection UI is constructed with the follow/following list of the user sending the message. |
onComplete | This is a callback to be called when message sending is completed or fails. |
DirectMessagePopupState
Response | Description |
---|---|
Cancel | When the pop-up is manually closed or if the sending fails. |
SendComplete | When the send request is completed and it closes. |
Situation by ErrorCode
Response | Description |
---|---|
31002 | This occurs if at least one incorrect UserID (an UserID that does not exist) is included when entering the user ID list in the userIds parameter. |
37011 | This occurs when you try to send a message while under a guest account. |
8001 | This happens when you continuously send the same message repeatedly. It can be sent once every 10 seconds. |
2001 | This occurs when the message content in the ‘Send’ field is either empty, contains only a space, or exceeds 1000 characters. |
0 | Network connection error cases such as network disconnection, connection instability, and other general error situations. |
-1 | Unidentifiable error. |
Usage example
Enter the sender directly and send
- Please add the Send DM Button to Canvas.
- Please write the example code as below.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
import { SocialService, DirectMessagePopupState } from 'ZEPETO.Module.Social';
import { Button } from "UnityEngine.UI";
import { WorldService } from 'ZEPETO.World';
export default class SendDirectMessage extends ZepetoScriptBehaviour {
// Variable to store the direct message
public message: string;
// Array of user IDs to send the direct message to
public userIds: string[];
// Reference to the button UI component
public sendDMButton: Button;
Start() {
this.sendDMButton.onClick.AddListener(() => {
// Check whether my id is included in the userIds array and return if it is included
if (this.userIds.includes(WorldService.userId)) {
console.log("userIds contains my userId");
return;
}
// Open a popup to send a direct message using the SocialService
SocialService.OpenPopupDirectMessage(this.message, this.userIds, (popupState: DirectMessagePopupState) => {
// Check the state of the direct message popup
if (popupState === DirectMessagePopupState.Cancel) {
console.log("You closed the pop-up or failed to send the message");
} else if (popupState === DirectMessagePopupState.SendComplete) {
console.log("The DM message was sent successfully, and the pop-up closed.");
}
});
});
}
}
- After writing the script, return to the Unity editor and drag the button in the inspector to set it. Enter the message body and the target user’s UserID for the message.
- Up to 20 people will be displayed in the UI, so enter up to 20 people.
- When you press the Send DM Button, the friend list UI appears.
- Select the target user and click Send.
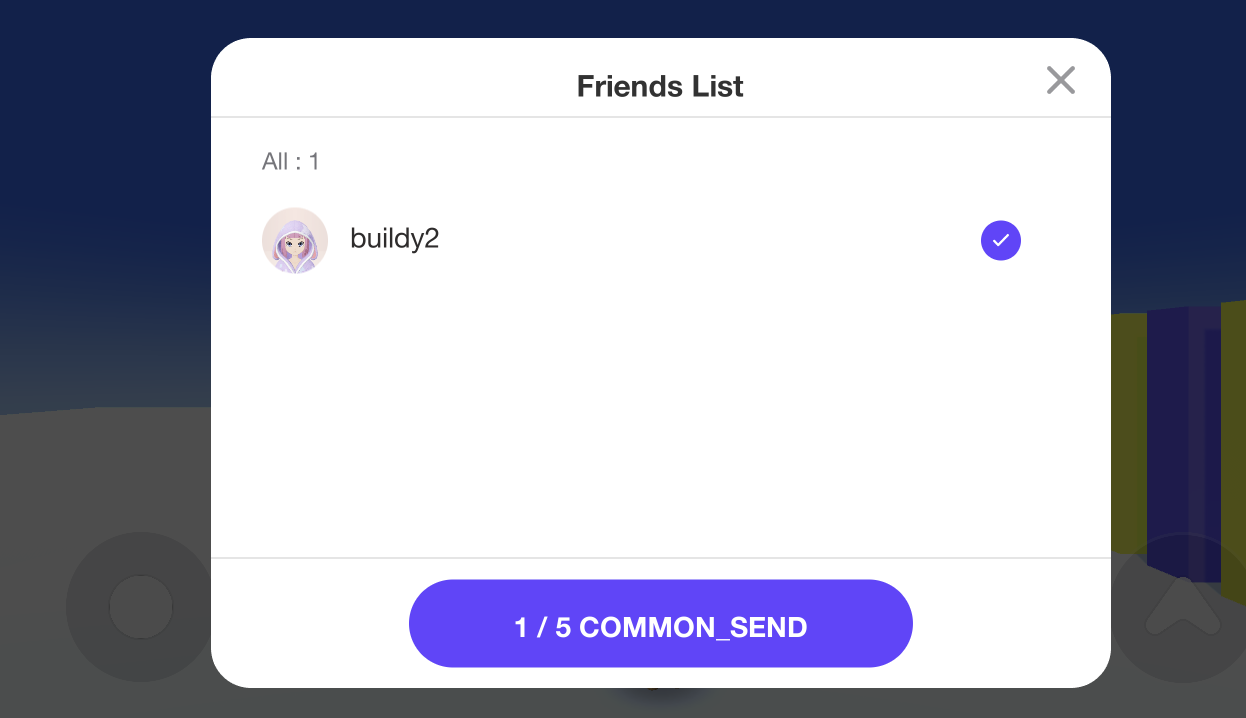
- If sending DM is successful, a Toast UI will appear indicating success, and a log will be output by the example script.
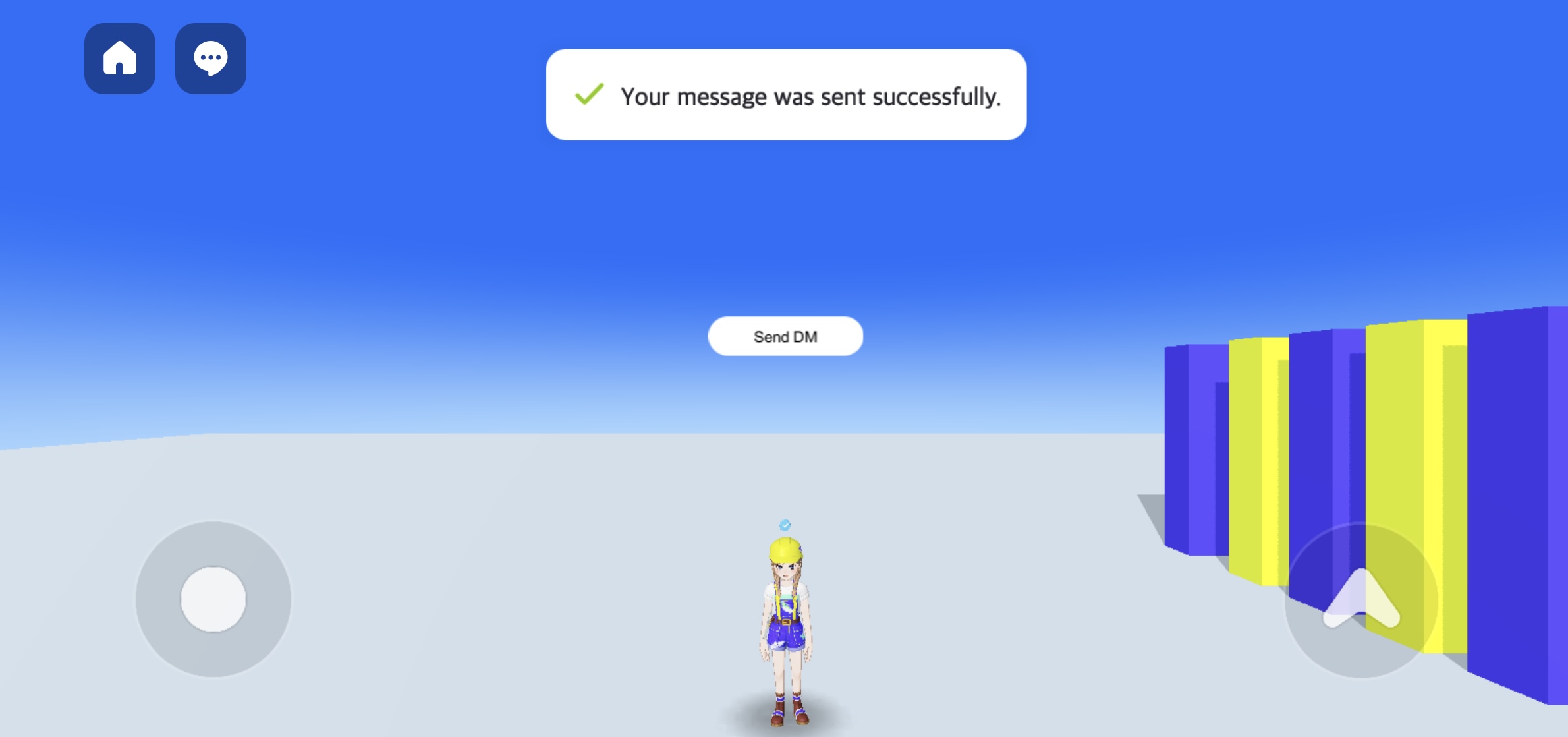
- Also, a DM has arrived for targetUser.
When testing in a pre-launch world, only the message is sent.
When using the DM sending API in a launched world, the world link is also sent along.
- If an error occurs, such as sending messages continuously or the message content is empty, the DM transmission will be processed as a failure.
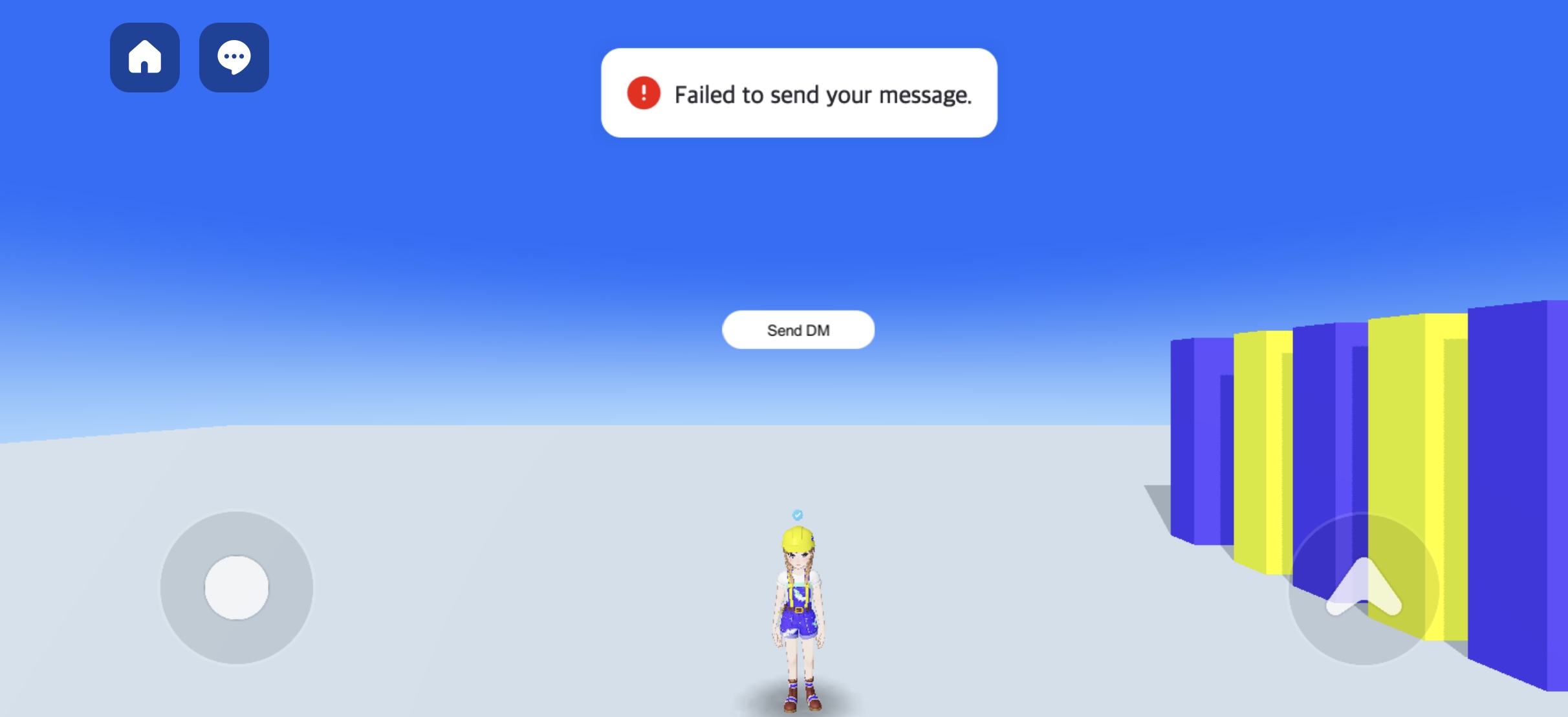
Send using following/follower list UI
- Please add a Button to the Canvas.
- Please write the example code as below.
- If you pass an empty list to the targetUserID parameter, the following/follower list UI will appear.
import { ZepetoScriptBehaviour } from 'ZEPETO.Script';
import { SocialService, DirectMessagePopupState } from 'ZEPETO.Module.Social';
import { Button } from "UnityEngine.UI";
export default class SendDirectMessage extends ZepetoScriptBehaviour {
// Variable to store the direct message
public message: string;
// Reference to the button UI component
public sendDMButton: Button;
// Private variable to store an array of user IDs, initialized as null
private emptyIds: string[];
Start() {
this.sendDMButton.onClick.AddListener(() => {
// Open a popup to send a direct message using the SocialService
// Use the class's message content and an empty array of user IDs
SocialService.OpenPopupDirectMessage(this.message, this.emptyIds, (popupState: DirectMessagePopupState) => {
// Check the returned state of the direct message popup
if (popupState === DirectMessagePopupState.Cancel) {
console.log("You closed the pop-up or failed to send the message");
} else if (popupState === DirectMessagePopupState.SendComplete) {
console.log("The DM message was sent successfully, and the pop-up closed.");
}
});
});
}
}
- After writing the script, return to the Unity editor and drag the button in the inspector to set it. Then please enter the body of the message.
- When you press the Send DM Button, the Following/Follower UI appears.
- Select the target user and click Send. (Up to 5 people can be selected)
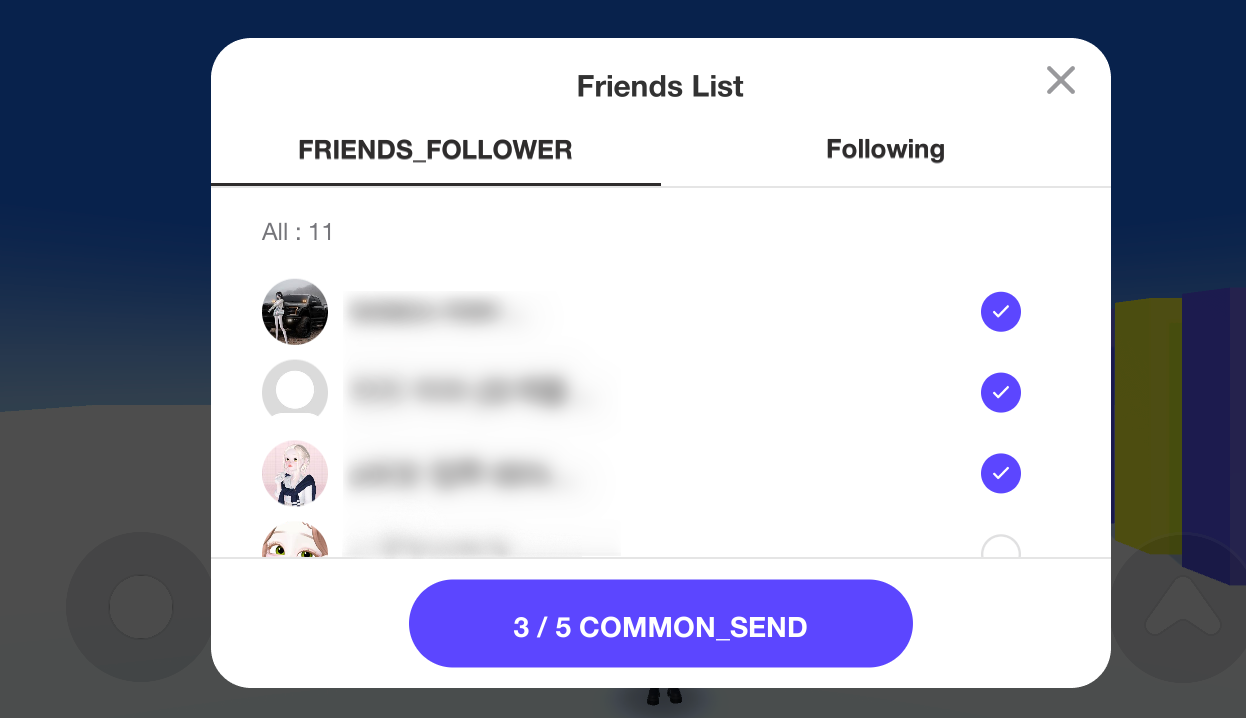
Caution
- You can send DM to up to 5 people at a time.
- You can’t send a DM to yourself.
- The actual number of following/following users may differ from the number of users displayed in the Send DM pop-up.
- This is because the users that can be selected are filtered and displayed.
- If you select a user who has blocked receiving DMs, it will be treated as a failure and DMs will not be sent.
- If you call the Send DM API frequently, it will be restricted and transmission will fail.
- You can send once every 10 seconds. Please be careful not to send indiscriminate DMs.
- If the message you send contains a URL, it will not work properly, so sending messages containing URLs is prohibited.
- If the message being sent is blank or empty, it will be treated as a failure. Please be sure to write the content of the message.